c# app推送消息
- 行业动态
- 2025-02-16
- 3582
在C#中实现App推送消息的功能,通常涉及使用第三方推送服务,如Firebase Cloud Messaging (FCM)、Apple Push Notification service (APNs)等,以下是使用FCM实现推送消息的详细步骤:
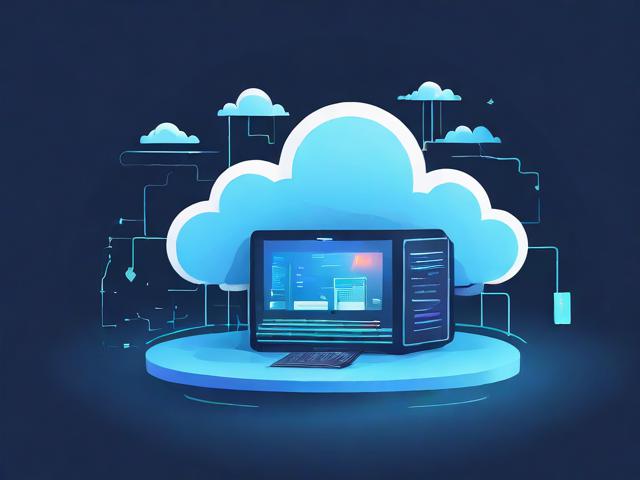
一、准备工作
1、注册项目:在Firebase控制台注册你的项目,并添加你的应用。
2、配置Android和iOS:下载google-services.json
(对于Android)和GoogleService-Info.plist
(对于iOS),并将它们添加到你的项目中。
3、安装依赖:确保安装了必要的NuGet包,如Xamarin.Firebase.Messaging
。
二、服务器端设置
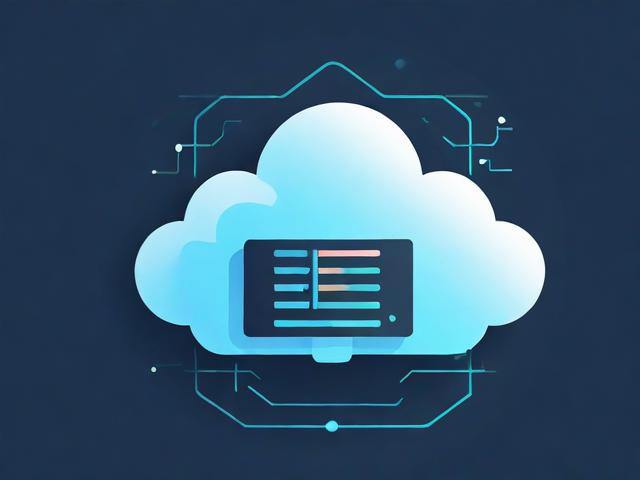
1、创建服务器密钥:在Firebase控制台中,导航到“项目设置”>“云消息传递”>“凭据”,然后创建一个新的私钥,下载生成的JSON文件,并将其保存在服务器上。
2、编写服务器端代码:使用以下C#代码示例向特定设备发送推送通知。
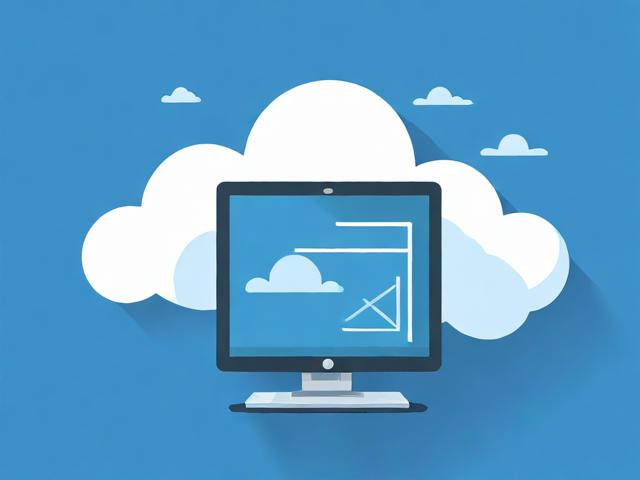
using System; using System.Net.Http; using System.Text; using System.Threading.Tasks; using Newtonsoft.Json.Linq; class Program { private static readonly string ServerKey = "YOUR_SERVER_KEY"; private static readonly string SenderId = "YOUR_SENDER_ID"; private static readonly string DeviceToken = "DEVICE_TOKEN"; static async Task Main(string[] args) { var message = new { to = DeviceToken, notification = new { title = "Hello World", body = "This is a test message" } }; var json = Newtonsoft.Json.JsonConvert.SerializeObject(message); var data = new StringContent(json, Encoding.UTF8, "application/json"); using (var client = new HttpClient()) { client.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Bearer", ServerKey); var response = await client.PostAsync($"https://fcm.googleapis.com/v1/projects/{SenderId}/messages:send", data); var result = response.Content.ReadAsStringAsync().Result; Console.WriteLine(result); } } }
将YOUR_SERVER_KEY
、YOUR_SENDER_ID
和DEVICE_TOKEN
替换为你的实际值,这段代码会向指定的设备发送一条包含标题和正文的推送通知。
三、客户端设置
1、Android:在MainActivity.cs
中配置Firebase App。
using Android.App; using Android.OS; using Firebase.Messaging; [Activity(Label = "@string/app_name", Theme = "@style/MainTheme", MainLauncher = true, ConfigurationChanges = ConfigChanges.ScreenSize | ConfigChanges.Orientation)] public class MainActivity : Activity { protected override void OnCreate(Bundle savedInstanceState) { base.OnCreate(savedInstanceState); Xamarin.Essentials.Platform.Init(this, savedInstanceState); FirebaseApp.InitializeApp(this); LoadApplication(new App()); } // 其他代码... }
2、iOS:在AppDelegate.cs
中配置Firebase App。
using UIKit; using Firebase.Core; using Firebase.Messaging; [Register("AppDelegate")] public partial class AppDelegate : global::Xamarin.Forms.Platform.iOS.FormsApplicationDelegate { public override bool FinishedLaunching(UIApplication app, NSDictionary options) { Firebase.Core.App.Configure(); // 其他代码... return base.FinishedLaunching(app, options); } // 其他代码... }
3、处理消息接收:实现IOnMessageReceived
接口来处理接收到的消息。
using Android.Gms.Common; using Android.Runtime; using Firebase.Messaging; using Xamarin.Forms; [assembly: Dependency(typeof(FirebaseMessagingService))] namespace YourNamespace.Droid { [IntentFilter(new[] { Gms.Common.Constants.ACTION_LOGIN })] [Service] public class FirebaseMessagingService : FirebaseMessagingService, IOnMessageReceived { const string TAG = "MyFirebaseMsgService"; public override void OnMessageReceived(RemoteMessage message) { SendNotification(message); } void SendNotification(RemoteMessage message) { var intent = new Intent(this, typeof(MainActivity)); intent.AddFlags(ActivityFlags.ClearTop); foreach (var data in message.Data) { intent.PutExtra(data.Key, data.Value); } PendingIntent pendingIntent = PendingIntent.GetActivity(this, 0, intent, PendingIntentFlags.OneShot); var notificationBuilder = new NotificationCompat.Builder(this, Resource.String.default_notification_channel_id) .SetContentTitle(message.GetNotification()?.Title) .SetContentText(message.GetNotification()?.Body) .SetSmallIcon(Resource.Drawable.ic_launcher) .SetAutoCancel(true) .SetContentIntent(pendingIntent); var notificationManager = GetSystemService(Context.NotificationService) as NotificationManager; notificationManager.Notify(0, notificationBuilder.Build()); } } }
四、FAQs
问题1:如何确保推送消息的安全性?
回答:为了确保推送消息的安全性,应采取以下措施:使用安全的连接(如HTTPS)传输消息;对敏感数据进行加密处理;验证消息的来源和完整性,防止消息被改动或伪造;限制消息的访问权限,确保只有授权的用户或设备才能接收消息,定期更新和审查安全策略也是保障推送消息安全的重要措施。
问题2:如何处理推送消息中的敏感信息?
回答:在处理推送消息中的敏感信息时,应遵循最小化原则,只发送必要的敏感信息,并在传输过程中进行加密处理,接收端应采取相应的安全措施来保护敏感信息,如使用安全的存储方式、限制访问权限等,还应遵守相关的隐私法规和合规要求,确保敏感信息的处理符合法律标准。
小编有话说
通过C#实现App推送消息功能可以大大提高用户参与度和留存率,无论是用于发送促销信息、新闻更新还是个性化通知,推送消息都是与用户保持联系的有效方式,希望本文能帮助你顺利实现这一功能,祝你开发顺利!