如何在服务器上添加源码?
- 行业动态
- 2025-01-10
- 4586
在服务器上添加源码是一个涉及多个步骤的过程,具体操作可能会因服务器环境、操作系统以及源码类型等因素而有所不同,以下是一个通用的指南,旨在帮助您将源码成功部署到服务器上:
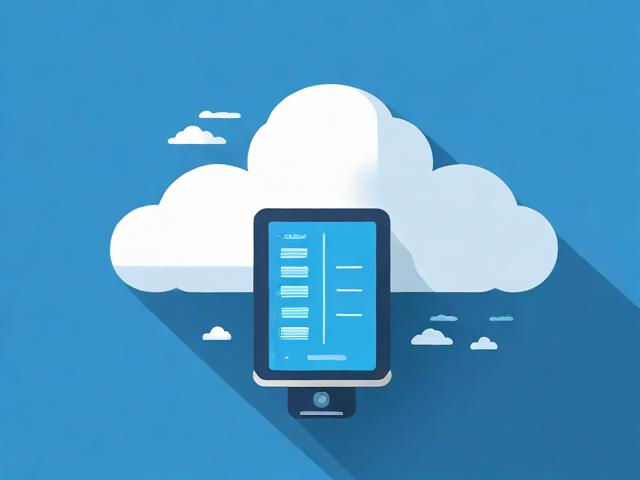
一、准备工作
1、确保服务器环境符合要求:在开始之前,请确保您的服务器环境(如操作系统、软件版本等)满足源码运行的要求。
2、获取源码:从可靠的来源下载源码文件,并确保文件完整且未被改动。
二、连接服务器
1、使用SSH登录服务器:打开终端或使用SSH客户端(如PuTTY),输入服务器的IP地址和登录凭证进行连接。
三、上传源码
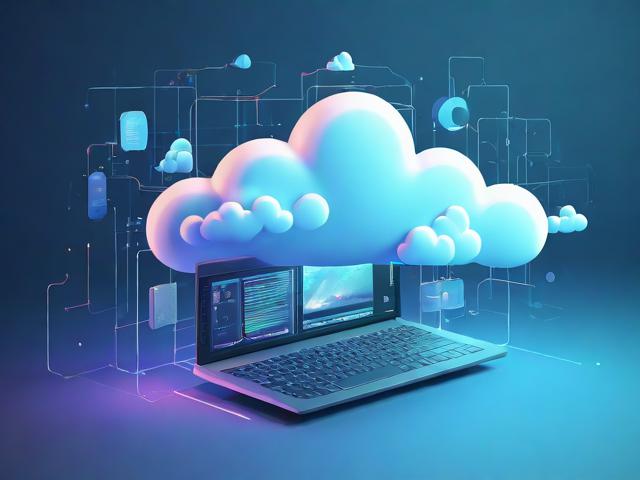
1、选择上传方式:根据个人喜好和服务器配置,选择合适的上传方式,如FTP、SCP或Git。
2、执行上传操作:
FTP:使用FTP客户端软件(如FileZilla)连接到服务器,并将源码文件拖拽到服务器目录中完成上传。
SCP:在本地计算机上使用SCP命令将源码文件上传到服务器指定目录。
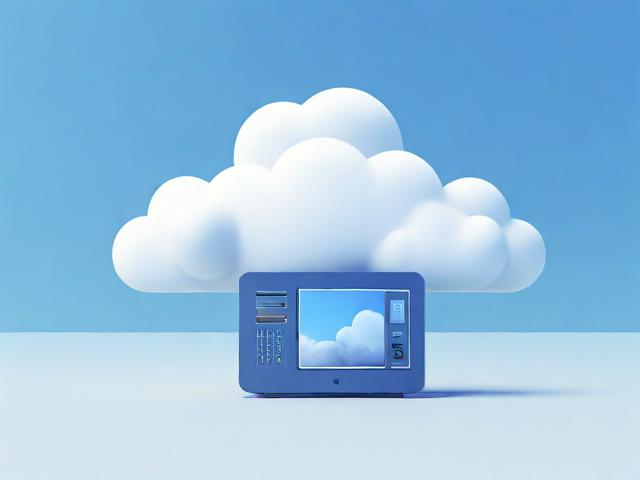
Git:如果使用Git进行版本控制,可以在本地将代码提交到远程仓库,然后在服务器上拉取最新代码。
四、解压与放置源码
1、导航到源码目录:使用cd命令进入存放源码的目录。
2、解压源码:如果源码是压缩文件,使用相应的解压命令(如unzip、tar)将其解压缩。
3、放置源码:将解压后的源码文件放置在Web服务器的根目录下的public_html或www文件夹中,以便通过浏览器访问。
五、安装依赖项与配置环境
1、安装必要的软件和工具:根据源码需求,在服务器上安装所需的软件和工具,如Web服务器(Apache、Nginx)、数据库服务器(MySQL、PostgreSQL)等。
2、安装依赖库:使用包管理工具(如apt、yum、npm等)安装源码所依赖的库或框架。
3、配置环境变量:如果需要,配置环境变量以确保源码能够正确执行。
六、配置源码
1、编辑配置文件:根据源码文档,编辑配置文件以设置数据库连接信息、API密钥等参数。
2、导入数据库(如果适用):如果源码依赖于数据库,需要在服务器上创建相应的数据库,并导入数据。
七、启动与测试
1、启动应用程序:根据源码类型,使用相应的命令或脚本启动应用程序。
2、测试功能:通过浏览器或其他网络工具访问服务器上的源码,测试其各项功能是否正常。
八、监控与维护
1、设置监控:配置监控工具以实时检测服务器和应用程序的运行状况。
2、定期维护:定期检查日志文件,处理可能出现的错误或异常,并更新源码以获取最新功能和安全补丁。
以下是两个关于服务器加源码的常见问题及其解答:
Q1: 如何更改服务器上的文件权限?
A1: 要更改服务器上的文件权限,可以使用chmod命令,要为所有文件和目录设置755权限,可以使用chmod -R 755 .
命令,请确保您有足够的权限来执行此操作,并且谨慎使用,以避免意外更改重要文件的权限。
Q2: 如果源码依赖于特定的软件或库,如何在服务器上安装它们?
A2: 要在服务器上安装源码所依赖的软件或库,您可以使用适当的包管理工具,对于Linux系统,常见的包管理工具包括apt(用于Debian/Ubuntu)、yum(用于CentOS/RHEL)和npm(用于Node.js),确定您需要安装的软件或库的名称,然后使用相应的包管理工具进行安装,在Debian/Ubuntu上,您可以使用sudo apt-get install package_name
命令来安装软件包,如果您不确定如何安装某个特定的软件或库,请查阅相关的文档或在线资源以获取帮助。
在服务器上添加源码是一个多步骤的过程,需要仔细规划和执行,通过遵循上述步骤和注意事项,您可以成功地将源码部署到服务器上,并通过浏览器或其他网络工具进行访问,请记得定期维护和更新您的服务器和源码,以确保其安全性和稳定性。